Scheduled Apex has long been key for updating records, and creating records on a scheduled basis on mass. Some of these things like updating several records at the same time can be tackled with scheduled flows. It is a powerful tool to have at your disposal with this in mind. I’m going to do a short tutorial on it.
Apex Scheduled Class
So I have written a scheduled apex class. The which will update the close date to a week time because the close date is passed already and it hasn’t been closed lost or won. I’ve included it below in the code block.
global class CloseDateIncrease implements Schedulable{
date TODAY = System.today();
date NEXTWEEK = TODAY.addDays(7);
global void execute(SchedulableContext sc){
List<Opportunity> lstofOpps = [SELECT Id, CloseDate FROM Opportunity WHERE IsClosed = FALSE AND CloseDate <= TODAY LIMIT 200];
List<Opportunity> lstofupdatedOpps = new list<Opportunity>();
if(!lstofOpps.isEmpty()){
for(Opportunity Opp : lstofOpps){
lstofupdatedOpps.add(Opp);
Opp.CloseDate = NEXTWEEK;
}
UPDATE lstofupdatedOpps;
}
}
}
This class is a lot simpler than it may read we are creating a list called lstofOpps. This list contains Id and Close Date where the stage isn’t closed and the Close Date is less than or equal to today. I’m limiting this to 200. Next, we are passing the Opportunities to a second list and updating the Close Date to next week.
Flow Replacement
Set up Schedule-Triggered Flow.
Create a new flow and select Scheduled-Triggered Flow.
- Click Set Schedule
- Set Date To Today
- Next set Time to an hour from now(So we have time to build it)
- Lastly set Frequency to Once (Can change this to daily after)
Now we have the scheduled part of the flow set up move on to the Choose Object select Opportunity and set IsClosed Equals False.
Create Formulas
We will create two formulas one called TODAY and one called NEXTWEEK. Both are date formula types.
TODAY Formula
You could hardcode the date values in this but what happens if you want to run this tomorrow you would need to change the value. Unfortunately, you can’t use formulas in the trigger criteria.
TODAY()
NEXTWEEK Formula
Uses the base TODAY() function and adds 7 to this which pushes it on a week.
TODAY() + 7
Update Records
- Set Use the opportunity $Record global variable
- Now set Criteria to CloseDate Less than or Equals to TODAY formula
- Set Close Date field to NEXTWEEK formula
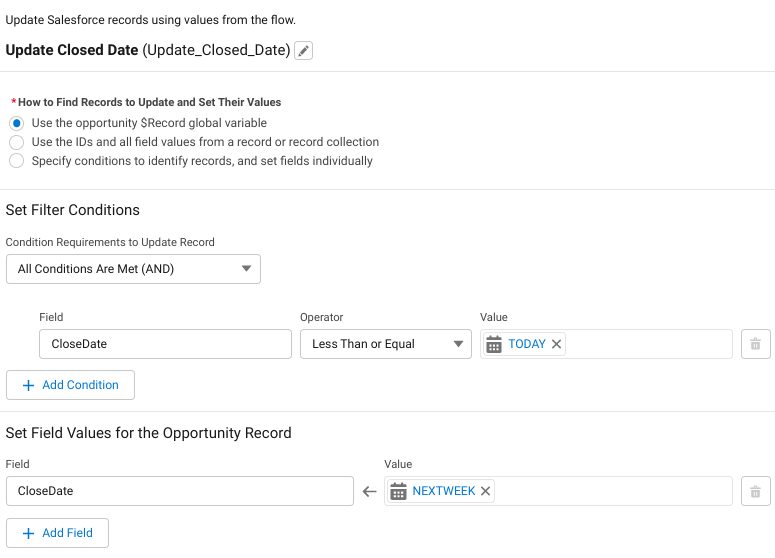
This is all you need to do because you have set a Criteria for updating you don’t need a decision if you want it to do something if it didn’t need updating it would do something else instead. This is now ready to test out. So make sure you have some Opportunities that have a Close Date of Today and Earlier and some that don’t.
Alternative Way Formula Field On Opportunity
Instead of creating a formula on that criteria, you could get around this by the following method. Create a formula field on an opportunity with a type of Boolean. Put the following in the formula field name the formula CloseDateToUpdate.
IF(CloseDate <= TODAY(),TRUE,FALSE)
Then you could call this directly in the object setup so the criteria would be if isClosed is False and if CloseDateToUpdate is True.
For other Apex Related replacements check out the beginner-friendly section on this site. For a larger project check out distribution and one page projects for something you can work through quickly.